Type Systems & Type Checking
Dynamic Type and Static Type
- Runtime vs Compile time
- Slow vs fast
- flexible vs inflexible
Strong Typing
- Sound type system: guarantees that a no type errors can occur at runtime
- cons
- Some checks can only be done dynamically (Array bounds checking)
- Such checking can cause serious performance degradation
- Even so-called strongly typed languages often have “holes" in the type system (e.g. variant records in Pascal)
Type Equivalence
- If types are required to match, the question of what types are considered to be “equivalent" arises
- Structural equivalence requires equivalent structures
- Identical basic types
- Same type constructor applied to equivalent types
- Name equivalence considered types to be equal only if the same names are used
- C uses structural equivalence except for records
Type Signatures
- The signature of a procedure is a description of the types of its arguments and type of its result.
- i.e) sqrt : real -> real, mod: integer X integer -> integer
Polymorphic Procedures
- A procedure that has multiple type signatures is called polymorphic
Failure Of Type Checking Due To Aliasing, Variant Records, Subroutine Calls
Citation:
-
Aho, Lam, Sethi, & Ullman, Compilers: Principles, Techniques, and Tools
-
UT Austin CS375: Compilers by G.Novak.
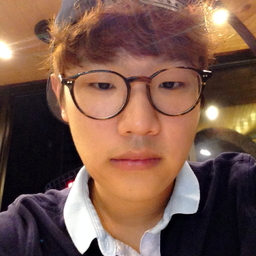
Posted on May 11th, 2015
Record Structure Declarations and References
What Information Is Stored In Symbol Table From Declaration
- A record declaration has a form such as : record $field_1$, …, $field_n$ : $type_1$ ; … end
-
- Initialize offset within the record to be 0.
-
- For each entry group,
- (a) Find the symbol table entry for the type
- (b) Allocate storage within the record using the storage allocation algorithm
- (c) Make a symbol table entry for each field, filling in its print name, type, offset in the record, and size
- (d) Link the entries for the fields to an entry for the record
-
- The size of the record is the total size given by the storage allocation algorithm, rounded up to whole words, (e.g. multiple of 8)
-
- Variant records simply restart the storage allocation at the place where the variant part begins. Total size is the maximum size of the variants
Generation Of Intermediate Code For References To Records
type date = record mo : 1..12;
day : 1..31;
year : integer end;
person = record name : alfa;
ss : integer;
birth : date end;
var people : array[1..100] of person;
- people[i].birth.day
- people[i] : (aref people (i-1)32 ) = (aref people (+ -32 ( i 32) ) )
References Using Pointers
Records With Variant Parts
Citation:
-
Aho, Lam, Sethi, & Ullman, Compilers: Principles, Techniques, and Tools
-
UT Austin CS375: Compilers by G.Novak.
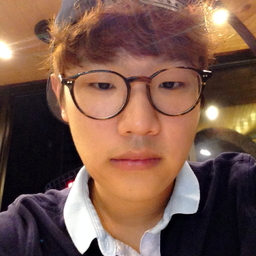
Posted on May 11th, 2015
Array References:
Methods For Storing Arrays
Effect of Array Order and Loop Order On Efficiency
Computation Of Effective Address(Formula)
Why Optimization Of Array References Is Important
Citation:
-
Aho, Lam, Sethi, & Ullman, Compilers: Principles, Techniques, and Tools
-
UT Austin CS375: Compilers by G.Novak.
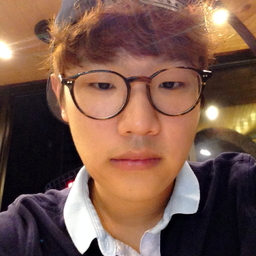
Posted on May 11th, 2015
Topics In Parsing Programming Language Constructs:
Current Status Variable
Type Coercion
- When a binary operator is reduced, it is necessary to check the types of the arguments, possibly to coerce an argument to the correct type, and to infer the result type.
- char < int < float < double
- For most operators, right-most type takes precedence
- For assignment, left-most type takes precedence
Conversion of Names With Constant Compile-Time Values To Constants
Intermediate Code for Non-Arithmetic Statements
Citation:
-
Aho, Lam, Sethi, & Ullman, Compilers: Principles, Techniques, and Tools
-
UT Austin CS375: Compilers by G.Novak.
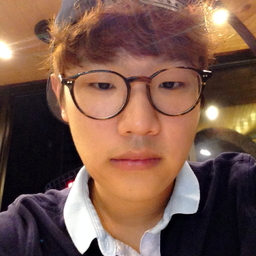
Posted on May 11th, 2015
Example Questions For Final Exam
Adderessing
From Midterm!
Show how an operator precedence parser would parse the string (ex. A-(B/C-D)/E+F)
- Show the contents of the stacks at each step; produce a tree as output
Give one advantage and disadvantage of hashing as a method of symbol table organization
Consider the regular expression (a|b)b+b. What is the simplest regular expression that denotes the same language?
Give the allowable form of productions for a Regular grammar.
Consider the following grammar :
- S -> a S
- S -> S b
- S -> b
- What kind of grammar is this in the Chomsky hierarchy?
- What kind of language does it denote?
- Is there a simpler kind of grammar that denotes the same language? If so, give the grammar, if not, explain why not.
Briefly and clearly define the following terms : vocab
Other Questions
A robot moves on a square grid. The robot can go forward, turn left or turn right. Give a grammar to describe the language of all sequences of moves that leave the robot pointing in the same direction as when it started
What kind of grammar is the above(in the Chomsky hierarchy)?
Describe a kind of local optimization
Describe what it means for a subexpression to be (a) available (b) busy (c) killed
Describe sources of extra run-time overhead in (a) time and (b)space in an OOP language
Draw boxes around the following code to show the basic blocks:
n := k*2;
if n < j then write(‘less’) else begin k := k - 1; write(‘more’) end;
writeln(k);
- Number the blocks and draw a flow graph; give the matrix form of the flow graph and its transitive closure
Give an advantage and a disadvantage for (a) call by reference (b) call by value
What are the most important things to optimize in a scientific program? Why?
Give three examples of computer architecture innovations that require compiler innovations to be effective
Briefly and clearly define the following terms: … 20 terms chosen from the vocabulary list on the study guide
Citation:
-
Aho, Lam, Sethi, & Ullman, Compilers: Principles, Techniques, and Tools
-
UT Austin CS375: Compilers by G.Novak.
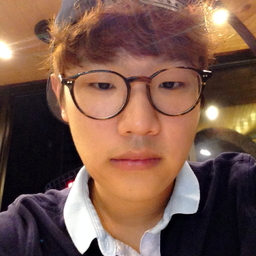
Posted on May 11th, 2015
왜 항상 자바Java는 C++보다 느린가?
이 글은 Dejan Jelovic님의 글을 번역 한 글입니다. 수 많은 오역이 있을 수 있습니다. 피드백 부탁드립니다!
“자바Java는 높은 능률Performance을 가졌다. 그럭저럭 높은 능률. 그리고 그럭저럭 이란 느림을 뜻한다."
Mr. Bunny( http://www.mrbunny.com/ )
자바Java 프로그래밍을 해본 사람이라면, 자바Java로 짜여진 프로그램들은 C++로 짜여진 프로그램보다 느린 걸 안다. 이것은 자바Java를 사용하는 사람들이 받아들여야 하는 인생의 진리이다.
그러나 많은 프로그래머들은 이 것이 임시적인 상황일 것이라고 자위하고, 남들을 설득하곤 한다. 그들은 자바Java가 느리게 만들어진 것이 아니라고 한다. 대신에 오늘날의 JIT는 상대적으로 나온지 얼마 안되었고, 최적화가 잘 안되었기 때문이라고.
그 말은 틀렸다. JIT가 얼마나 좋아지건 간에, Java는 항상 C++보다 느릴 것이다.
The Idea
자바가 C++만큼 빠르거나 더 빠르다고 하는 사람들의 생각은 이렇다. 더 통제되는 프로그래밍 언어는 컴파일러가 최적화할 가능성을 더 열어준다. 그래서 모든 코드를 손으로 최적화 할 것이 아니라면, 전체적으로 컴파일러는 더 좋은 성능을 보여줄 것이다.
이 말은 옳다. Fortran은 더 통제되는 언어이기 때문에 계산에 있어서 C++을 완전히 발라버린다. Pointer Aliasing의 걱정 없이 컴파일러는 최적화를 더 잘 해준다. C++이 Fortran의 속도를 흉내낼 수 있는 방법은 영리하게 잘 만들어진 Blitz++같은 라이브러리를 이용하는 것 뿐이다.
그러나 이런 결과를 가져다 주기 위해서 애초에 프로그래밍 언어는 컴파일러에게 최적화의 가능성을 열어 줄 수있게 만들어 졌어야 한다. 공교롭게도 자바Java는 이런 방법으로 만들어지지 않았다. 그래서 컴파일러가 얼마나 똑똑해 지건 간에 자바Java는 C++의 속도를 절대 따라잡지 못할 것이다.
The Benchmarks
거꾸로, 자바Java가 C++ 만큼은 빠를 수 있는 분야는 전형적인 벤치마크를 할 때이다. 만약 N번째 피보나치 넘버를 계산하거나 Linpack(벤치마크 방법)을 돌려야 한다면, 자바가 C++보다 느릴 이유가 전혀 없다. 모든 계산이 한 클래스 안에서 이루어지고, int나 double같은 기본형Primitive 데이터만 다룬다면 자바는 C++의 발자국들을 따라 잡을 수 있다.
The Real World
프로그램에 오브젝트를 사용하는 순간부터 자바는 최적화를 할 가능성을 잃어버린다. 다음에서 그 이유들을 다룰 것이다.
1. 모든 오브젝트는 힙Heap에 할당된다.
자바는 int나 double같은 기본Primitive 자료형만 스택Stack에 할당한다. 모든 오브젝트는 힙Heap에 할당된다.
Identity semantic을 가지는 큰 오브젝트들의 경우에는 문제가 안된다. C++ 프로그래머들도 이런 오브젝트는 힙에 할당한다. 그러나 성능을 저하시키는 주요 이유는 단순 값만 들고 있는 (Value semantics) 작은 오브젝트들의 경우이다.
이런 작은 오브젝트가 무엇인가? 나에게 이것들은 이터레이터들이다. 나는 그들을 내 디자인(코드)에 많이 쓴다. 어떤 사람들은 복잡한 숫자를 쓸 수도 있다. 예로 3D 프로그래머들은 벡터Vector나 포인트Point 클래스를 쓴다. 시간에 연계된 자료를 다루는 사람들은 Time클래스를 쓴다. 이런 자료형들을 쓰는 사람들은 비용 0의 스택할당 보다 상수 시간이 걸리는 힙 할당을 쓴다. 이 것들로 루프를 돌리면 O(n)과 상수 0의 차이가 난다. 다른 루프를 추가하면 O($n^2$)과 상수 0의 차이가 난다.
2. 엄청 많은 형변환Cast.
템플릿Template의 등장으로, 뛰어난 C++ 프로그래머들은 high-level 프로그램에도 형변환Cast를 최대한 피할 수 있었다. 공교롭게도 자바는 템플릿Template이 없고, 자바 코드는 형변환Cast으로 넘쳐난다.
이 것은 성능에 무슨 영향을 끼칠까? 자바의 모든 형변환은 동적Dynamic 형변환이고, 비싼 비용을 지불해야 한다. 얼마나 비싼지 동적 형변환을 예로 들어 보겠다.
가장 쉬운 예는 클래스에 번호를 할당하고, 두 클래스가 연관되었는지를 판단하는 행렬을 만들고, 만약 그렇다면 offset은 형변환을 하기 위해 포인터에 추가되어야 될 것이다. 그 경우에, 형변환을 위한 수도코드는 다음과 같다 :
DestinationClass makeCast (Object o, Class destinationClass)
{
Class sourceClass = o.getClass(); // JIT compile-time
int sourceClassId = sourceClass.getId(); // JIT compile-time
int destinationId = destinationClass.getId();
int offset = ourTable [sourceClassId][destinationClassId];
if (offset != ILLEGAL_OFFSET_VALUE)
{
return ;
}else throw new IllegalCastException();
}
작은 형변환을 위해 너무 많은 코드를 쓴다. 그리고 여기에 장밋빛 그림이 있다 - 클래스의 관계를 나타내기 위해 행렬을 쓰는 것은 너무 많은 메모리를 잡아먹고 미치지 않은 컴파일러라면 이 짓을 할리가 없다. 대신에 그들은 Map이나 상속 계층을 뒤질 것이다. - 이 두 경우에는 계산이 더 느리다.
3. 메모리 사용량의 증가
자바 프로그램들은 C++ 프로그램들이 데이터를 저장하는 것의 거의 2배를 사용한다. 이는 다음과 같은 세 가지 이유가 있다.
-
- GC를 켜놓은 프로그램들은 직접 손으로 메모리 관리를 하는 프로그램보다 메모리를 50% 정도 더 쓴다.
-
- C++에선 많은 오브젝트들이 스택에 할당 될 때, 자바에서는 힙에 할당 된다.
-
- 자바의 오브젝트는 더 큰데, 모든 오브젝트가 virtual table을 가지는 데다가 synchronization primitive를 지원하기 때문이다.
큰 메모리를 사용하는 프로그램들은 프로그램이 디스크에서 스왑 아웃될 확률을 증가시킨다. 그리고 스왑 아웃이 된다면, 속도는 말할 것도 없다.
4. 디테일을 다루는 컨트롤의 부재
자바는 의도적으로 심플한 언어로 설계되었다. C++은 프로그래머에게 디테일을 다룰 수 있는 많은 기능들을 제공한다. 이 기능들은 자바에서 의도적으로 삭제되었다.
예를 들어 C++은 Locality of reference를 향상시킬 수 있는 기능을 제공한다. 많은 오브젝트들을 한 번에 할당하고, 해제하는 기능도 제공한다. 포인터로 멤버를 빠르게 접근할 수 있는 트릭도 제공한다...
하지만 이런 기능들을 자바에 없다.
5. High-level 최적화의 부재
프로그래머들은 high-level 개념들을 다룬다. 반대로 컴파일러는 low-level만을 다룬다. 프로그래머에게 Matrix라고 이름지어진 클래스는 Vector라고 이름 지어진 클래스와 다른 high-level 개념이다. 컴파일러에게 이들은 심볼 테이블Symbol Table의 일부일 뿐이다. 컴파일러가 신경쓰는 것은 그 클래스가 가지는 함수들과, 그 함수들 안의 구문들 뿐이다.
이제 이걸 생각해보자 : $x^y$를 반환하는 exp(double x, double y)라는 함수를 사용한다고 생각해보자. 컴파일러는 이 구문들을 들여다 보는 것만으로 exp(exp(x,2), 0.5)가 x로 최적화 될 수 있는 걸 알 수 있을까? 당연히 아니다.
컴파일러가 할 수 있는 모든 최적화는 구문 단계에서 이루어지며, 그들은 컴파일러 안에 쓰여져 있다. 위의 예제에서 가 exp(exp(x,2), 0.5)가 x와 같다는 것을 알거나 코드 안에서 함수를 부르는 순서가 잘못되었다는 걸 프로그래머는 안다. 하지만 컴파일러는 이걸 구문 레벨에서 뜯어낼 수 없기 때문에, (컴파일러가 구문 단계에서 이걸 뜯어 낼 수 있게 되기 전까지는) 최적화는 되지 않을 것이다.
그래서 만약 high-level 최적화가 되길 바란다면, 프로그래머가 컴파일러에게 high-level 최적화 조건들을 알려줄 수 있는 방법이 있어야 한다.
유명한 프로그래밍 언어와 시스템 중 이런 방법을 가진 것은 없다. 그러나 C++에서는 템플릿 메타프로그래밍으로 high-level 오브젝트들의 최적화를 해낼 수 있다. Temporary elimination, partial evaluation, symmetric function call removal 그리고 더 많은 다른 최적화들은 템플릿을 이용함으로써 구현할 수 있다. 당연히 모든 high-level최적화가 이런 방식으로 되는 것은 아니다. 그리고 이런 것들을 사용하는 것은 어렵고 귀찮다. 그러나 상당히 많은 수가 해결될 수 있으며, 사람들을 이미 이런 기술을 이용해 snazzy libraries를 구현 했다.
다시 한 번 공교롭게도, 자바에는 메타프로그래밍 설비가 되어있지 않다. 그러므로 (이를 이용한) high-level 최적화는 자바에서 불가능하다.
So...
지금까지의 기능을 볼 때 자바는 결코 C++처럼 빠를 수 없다. 이 것은 곧 자바가 고성능 소프트웨어나 COTS arena에 적당하지 않다는 것을 의미한다. 그러나 자바는 배우고 쉽고, 관대하고, 이용하기 쉬운 방대한 라이브러리가 있어서 작거나 중간 정도의 크기의 프로그램을 만드는 데 적당하다.
역주
정확한 번역보다 읽기 쉽게 의역하려고 노력 했습니다.
번역 : 정성원(sungpia@me.com)
Citation
- Jevolic, D. (n.d.). Why Java Will Always Be Slower than C. Retrieved April 15, 2015, from http://www.jelovic.com/articles/why_java_is_slow.htm
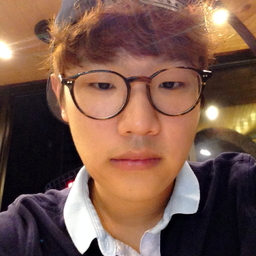
Posted on April 15th, 2015
OOP 인터뷰 질문 정리
What are the basic concepts of OOP?
- Abstraction
- Polymorphism
- Inheritance
- Encapsulation
What is dynamic or run time polymorphism?
Object와 Class의 차이
Class를 인스턴트화(Instantiation)하면 Object(Instance)가 된다.
객체는 클래스의 인스턴스다.
객체 간의 링크는 클래스 간의 연관관계의 인스턴스다.
실행 프로세스는 실행 프로그램의 인스턴스다.
추상적인 컨셉에서 논할 때는 클래스와 객체로 이야기 한다.
프로그램 컴파일 레벨의 아래 쪽에서 논할 때는 클래스와 인스턴스로 이야기 한다.
Object has states and behaviors. An object is an instance of class.
Class can be defined as a template/blue print that describes the behaviors/states that object of its type support.
오브젝트
오브젝트는 실 세계와 연관이 깊다. ‘개’라는 오브젝트를 우리는 실 생활에서 쉽게 찾을 수 있다.
클래스
클래스는 각 각의 오브젝트들을 만들기 위한 청사진이다.
Local variable, Instance variable, class variable.
$1 :
Instantiation
자바에서는 new 키워드를 사용하면 인스턴스화가 된다.
(declaration class_name var_name = instantiation class_name(initialization);
Method
메소드는 오브젝트와 오브젝트의 통신을 하는 방법이다.
오브젝트의 상태를 나타내는 것은 인스턴스 변수이다.
Abstract Class
Class/Static Method
Static/Class Initializer
Constructor
Destructor/Finalizer
Superclass /Baseclass
Subclass/Derived Class
Inheritance
Encapsulation
Polymorphism (예시 없이)
Multiple Inheritance
Delegation
Composition /Aggregation
Interface /Abstract class
Interface /Protocol
Method Overriding
Method Overloading
IS-A 와 HAS-A
Method Signatures
Method Visibility
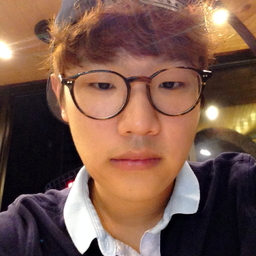
Posted on April 14th, 2015
HTTPS and SSL Certificate
HTTPS vs HTTP
HTTP stands for Hypertext Transfer Protocol. Which means, it is a protocol that we can use for transferring Hypertext datas. “S" in HTTPS stands for Over Secure Socket Layer. Intuitively, it’s secured HTTP protocol. HTTP sends data without encryption; hackers can easily eavesdrop data flowing between server and client.
HTTPS and SSL
HTTPS and SSL are not same concept as Internet and Web are not same. SSL protocol is more broad concept; HTTPS works on the SSL protocol.
SSL and TLS
Netscape developed SSL, but this term was redefined by IETF to TLS. However, no one cares and it is stilled called by SSL.
SSL Digital Certificate
Communication between client and server can be guaranteed by third person, and documented to SSL certificate. Right after client approaches server, server sends this certificate to client. Client examines whether is certificate is trustable and safe, then client processes next procedures. There we can earn benefits by SSL.
- Hide information from eavesdropper
- Client can determine if server is trustable or not
- Both can assure if communication was changed in the middle or not
Citation
http://opentutorials.org/course/228
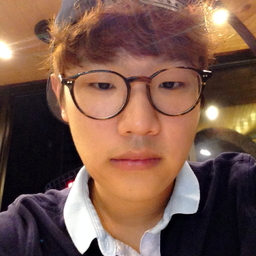
Posted on April 4th, 2015
Setting AWS for Who’s Hungry?
1 Load Balancer
1 Web/App Server with Passenger
1 DB server ( postgreSQL )
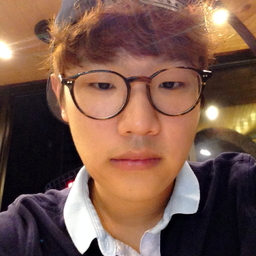
Posted on April 4th, 2015
Description of methods
Phone
Send Verification Message to User
- method : POST
- version : v1
- URL : /v1/phone/sendsms
Description
You can use this method for sending verification SMS to user, with 6 random digit code
Parameters
- contact
Constraints
- contact : should be “+15129994336" form
Response
Simple text, “SENT"
Verify 6 digit number from SMS
- method : POST
- version : v1
- URL : /v1/phone/verify
Description
You can use this method to verify SMS from our server.
Parameters
- contact
- code
Constraints
- contact should be “+15129994336" form.
Response
“OK" if right, or “Wrong" if wrong.
User
Creates User
- method : POST
- version : v1
- URL : /v1/users
Description
You can register user, using parameters above. This is exactly same as “Sign Up" that you can see everywhere on the website. You’ll use user_id from response for every single actions for Who’s Hungry? server
Parameters :
- name : name of the user from facebook
- picture : url of the profile picture from facebook
- contact : user’s contact number
- email : user’s email address
- fb_id : facebook id of the user
- access_token : access_token from Facebook login
- os_type : iOS / android
- push_id : push id of the device
Constraints
- contact
should be unique. If you try to make user with existing contact number, server will not allow any actions. you can use put method existing below.
Response
- id : this is user_id
- name
- picture
- contact
- fb_id
- created_at
- updated_at
- devices
- id : this is device_id
- user_id
- os_type
- push_id
- created_at
- updated_at
Show One User
- method : GET
- version : v1
- URL : v1/{token}/user/{user_id}
Description
Parameter
Constraint
Response
see apiary
Update User
- method : PUT
- version : v1
- URL : v1/{token}/users
Description
Parameter
Constraint
Response
- id : this is user_id
- name
- picture
- contact
- fb_id
- created_at
- updated_at
- devices
- id : this is device_id
- user_id
- os_type
- push_id
- created_at
- updated_at
Auth
login to the server
- method : POST
- version : v1
- URL : /v1/auth
Descriptions
If access_token from the Facebook is expired, you should update user cause Who’s Hungry? server will never allow you to login. (It will never match data from the server)
Response token will allow you every single authorization towards the server.
Parameters
- user_id : user_id of Who’s Hungry? server
- access_token : access_token from facebook
Constraints
none
Response
- user_id
- id
- login_type
- token
- created_at
- updated_at
Group
Create Group
- method : POST
- version : v1
- URL : /v1/{token}/groups
Descriptions
Creates group according to invitation. We invite through contact number, and
Parameters
- invitation
- fb_id or contact
- fb_id or contact
- fb_id or contact … (at least one contact number)
Constraints
- token
We need token to authorize user - contact
Contact number should contain country code, ex : “+15129994336".
Response
- id : id of group
- user_id : admin user_id
- name : name of the group
- created_at
- updated_at
- users
- id
- fb_id
- name
- picture
- contact
- created_at
- updated_at
- devices
- id : this is device_id
- user_id
- os_type
- push_id
- created_at
- updated_at
Show groups where you belongs
- method: GET
- version: v1
- url: v1/{token}/groups
Description
Get the list of groups that you’re belonging to
Parameters
none
Constraints
none
Responses
see apiary
Show one group
- method: GET
- version: v1
- url: v1/{token}/group/{group_id}
Description
Parameters
Constraints
Responses
Vote
Create Vote
- method : POST
- version : v1
- URL : /v1/{token}/{group_id}/votes
Description
Create “Vote" with information of votes, and known information with restaurants. Automatically creates “Choices" in the vote, and restaurants are automatically registered in the servers so you can use it.
Parameters
- vote_type : vote type such as “lunch", “dinner" ...
- name : name of the vote
- expires_in : integer minute, which is, vote will expire in 10 minute => 10
- expires_at : Datetime of expiring time of the vote
- restaurants : JSON array of restaurants
- place_id : id of restaurant(from google place API, yelp …)
- name : name of restaurant
- picture : url of profile pic of restaurant
- lat : latitude
- lng : longitude
- rating : official rating
Constraints
Response
- id : id of vote
- group_id : id of group
- vote_type
- name
- expires_in
- expires_at
- created_at
- updated_at
- choices
- id
- vote_id
- restaurant_id
- count
- created_at
- updated_at
Show one Vote
- method: GET
- version: v1
- URL: v1/{token}/vote/{vote_id}
Description
show one vote
Parameters
none
Constraints
none
Response
Choices
View all the choices in the vote
- method: GET
- version: v1
- URL: v1/{token}/{vote_id}/choices
Description
Look up all the choices that belongs to certain {vote_id}.
Parameters
none
Constraints
none
Response
- choices
- id
- vote_id
- restaurant_id
- count
- created_at
- updated_at
Create new choice in the vote
- method: POST
- version: v1
- URL: v1/{token}/{vote_id}/choices
Description
Create single choice under certain {vote_id}, which is adding a restaurant to vote.
Parameters
- place_id
- name
- picture
- lat
- lng
- rating
Constraints
Response
- id
- vote_id
- restaurant_id
- count
- created_at
- updated_at
Make a choice (make a vote)
- method: PUT
- version: v1
- URL: v1/{token}/choice/{choice_id}
Description
Make a vote, with the count with given {choice_id} from other APIS.
Parameters
- count : up vote? down vote?
Constraints
- count
should be {-1, 1}, one can remain in state {upvote, not voted, down vote }
Response
- id
- vote_id
- restaurant_id
- count
- created_at
- updated_at
Rsvp
Make a RSVP
- method: POST
- version: v1
- URL: v1/{token}/{vote_id}/rsvps
Description
Make a Rsvp, and send push notification between devices so RSVP of the vote can remain updated.
Parameters
- rsvp : integer (1: going, -1: not going, 0: default)
Constraints
Response
- id
- vote_id
- user_id
- rsvp
- created_at
- updated_at
Get one vote’s RSVP list
- method: GET
- version: v1
- URL: v1/{token}/{vote_id}/rsvps
Description
Get all RSVPs from Vote
Parameters
none
Constraints
Response
- rsvps : JSON array of rsvps
- id
- vote_id
- user_id
- rsvp
- created_at
- updated_at
Restaurant
Show one restaurant’s information
- method: GET
- version: v1
- URL: v1/{token}/restaurant/{restaurant_id}
Description
Get all the information about the restaurant. {restaurant_id} either can be Who’s Hungry? server’s id or place_id
Parameter
none
Constraints
Response
- id
- place_id
- name
- picture
- lat
- lng
- rating
- created_at
- updated_at
Location
Update user’s location
- method: POST
- version: v1
- URL: v1/{token}/locations
Description
Update user’s location
Parameters
- lat : latitude of the user
- lng : longitude of the user
- pickup : {1: “I want to be picked up", 2: “I am driving", 3: “I’m inviting you to my home"}
Constraints
Response
- id
- user_id
- lat
- lng
- pickup
- updated_at
- created_at
Get Vote's location
- method: GET
- version: v1
- URL: v1/{token}/{vote_id}/locations
Description
Get group’s location
Parameters
none
Constraints
“ I have a question :: Do we have to filter users that who RSVPed?"
Response
- locations
- id
- user_id
- lat
- lng
- pickup
- updated_at
- created_at
Lobby
See Lobby
- method : POST
- version : v1
- URL: v1/{token}/lobbies
Description
Get lobby
Parameters
- limit : limit of lobby {should send default :: 40}
- offset : starting point {should send default :: 0}
Constraint
please send limit and offset
Response
JSON array of votes : chk document for vote
Chat
upload your chat
- method : POST
- version: v1
- URL: v1/{token}/{vote_id}/chat
Description
Upload chat from the user to the server
Parameters
- text
Constraint
Response
See all Chats
- method : GET
- version: v1
- URL: v1/{token}/{vote_id}/chat
Description
See all the chats
Parameters
none
Contraint
Response
Overlap
Show one overlap
- method : GET
- version : v1
- URL: v1/{token}/{choice_id}/overlap
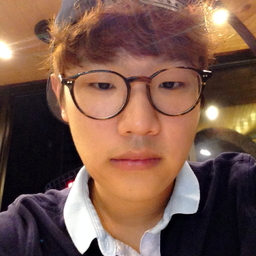
Posted on March 27th, 2015